The ActiveCampaign API provides developers with a powerful toolset to interact with the ActiveCampaign platform. In this blog, we will explore a few aspects of working with ActiveCampaign API, including authentication, pagination, ordering, filtering and data retrieval.
Authentication
To authenticate with the ActiveCampaign API, you will need to use an API key. All requests to the API are authenticated by providing your API key. By authenticating with the ActiveCampaign API using your API key, you gain the necessary access to leverage the platform's functionality and integrate it with your applications, systems, or workflows.
Generate an API Key
Log in to your ActiveCampaign account with your user name and password.
Your API key can be found in your account on the Settings page under the Developer tab. Each user in the ActiveCampaign account has their own unique API key.
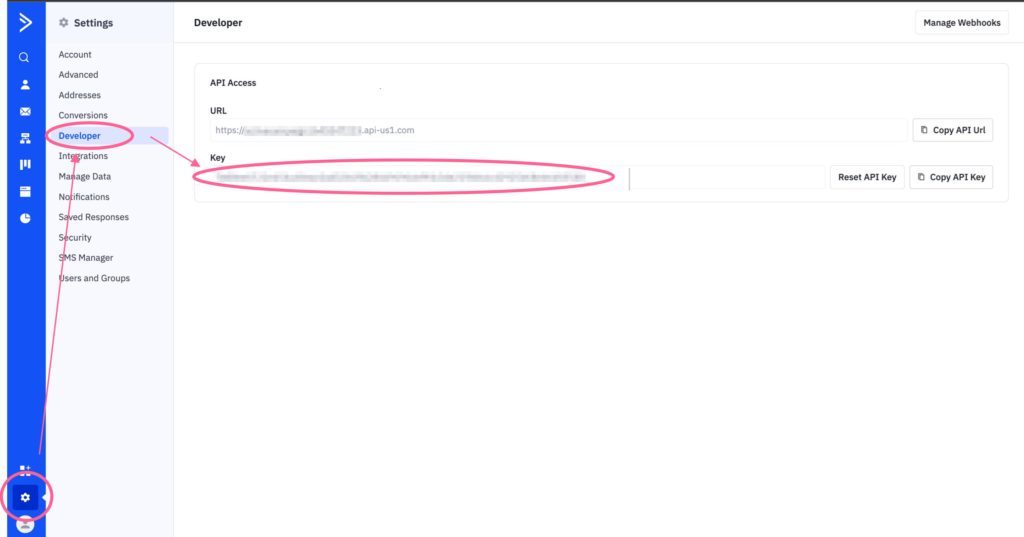
The API key should be provided as an HTTP header named Api-Token
. We will construct our headers to be as listed below:
headers = {
"accept": "application/json",
"Api-Token": keys.api_key
}
keys.py
is a python 'configuration file' that holds all our sensitive information such as the api_key
.
Base URL and End URL
The API is accessed using a base URL that is specific to your account. Base URL represents the root or starting point of the API. It specifies the domain and protocol (usually HTTP or HTTPS) where the API is hosted.
Generally, the URL will be in the form of https://<your-account>.api-us1.com
.
Your account name can be found in your account on the Settings page under the Developer tab.
An Endpoint URL is a specific URL that identifies a particular resource or operation provided by an API. It represents a unique location within the API's URL structure to access a specific functionality or retrieve specific data. An endpoint URL is appended to the Base URL to form a complete URL that can be used to make API requests.
Pagination
Pagination is a technique used to break down large result sets into smaller, manageable chunks or pages. It enables you to retrieve data in a controlled and efficient manner by fetching a subset of data at a time.
The ActiveCampaign API provides pagination parameters that allow Us to control the pagination of results when retrieving data. Using these pagination parameters, We can control the number of items per page, navigate through the result pages, and retrieve data in smaller, manageable chunks.
Parameter | Description |
---|---|
limit | The number of results to display in each page (default = 20; max = 100). |
offset | The starting point for the result set of a page. This is a zero-based index. For example, if there are 39 total records and the limit is the default of 20, use offset=20 to get the second page of results. |
Ordering
Ordering in the ActiveCampaign API allows us to specify the sorting order (ASC
or DESC
) for the returned results based on one or more attributes. It enables us to control the arrangement of the data based on a particular field or a combination of fields.
The order in which sorting criteria is applied reflects the order in which the parameters are set in the query string.
In the example below, we constructed the end url as: end_url = /api/3/contacts?orders[lastName]=ASC&orders[email]=DESC
, with the results first sorted by the contacts last name
, and then sorted by the contacts email address
.
Filtering
The filtering parameter in the ActiveCampaign API allows us to retrieve a subset of data that matches specific criteria. It enables us to narrow down the results based on one or more conditions, providing a way to query the API for data that meets certain requirements.
In the example below, we constructed the end url as: end_url = /api/3/campaigns?orders[lastName]=ASC&filters[type]=single
to get all single type campaigns in an ascending order.
Meta
Metadata can be represented as a top-level member named "meta". Any information may be provided in the metadata. Its most common use is to return the total number of records when requesting a collection of resources.
when constructing a request API call to get the first ever project, we also get the top-level meta
showing us the total objects in campaigns.
headers = {
"accept": "application/json",
"Api-Token": keys.api_key
}
base_url = "https://<your-account>.api-us1.com"
end_url = "/api/3/campaigns?limit=1&orders[sdate]=ASC"
r = requests.get(base_url + end_url, headers=headers)
data = r.json()
The last object for the above output is 'meta': {'total': '212'}
to inform us we have 212 campaigns in total.
Calculating #Pages
Let's construct a function to calculate the number of pages we have when listing our campaigns in ActiveCampaign. We will use the meta parameter for that.
def get_pages():
headers = {
"accept": "application/json",
"Api-Token": keys.api_key
}
base_url = "https://<your-account>.api-us1.com"
end url = "https://theinformationlabnl.api-us1.com/api/3/campaigns? orders[sdate]=DESC"
r = requests.get(url, headers=headers)
data = r.json()
cnt_campaigns = int(data['meta']['total'])
pages = int((cnt_campaigns/100)+1)
return pages
The get_pages()
function sends a GET request to the ActiveCampaign API to retrieve campaign data, calculates the total number of campaigns, and determines the number of pages required to retrieve all the campaigns based on a fixed page size of 100. The function then returns the total number of pages.
Get All Campaigns from ActiveCampaign
We will now connect to the API and get a list of all our campaigns.
def get_all_campaigns():
headers = {
"accept": "application/json",
"Api-Token": keys.api_key
}
base_url = 'https://theinformationlabnl.api-us1.com'
# Looping through pages
camps_list = []
for i in range(get_pages()):
offset = str(i*100)
end_url = f'/api/3/campaigns?limit=100&orders[sdate]=DESC&offset={offset}'
r = requests.get(base_url + end_url, headers=headers)
data = r.json()
# Looping through campaigns
for item in data['campaigns']:
int_camps_list = []
camp = {
'campaign': item['name'],
'c_date': item['cdate'],
'send_amt': item['send_amt'],
'total_amt': item['total_amt'],
'opens': item['opens'],
'uniqueopens': item['uniqueopens'],
'linkclicks': item['linkclicks'],
'uniquelinkclicks': item['uniquelinkclicks'],
'subscriberclicks': item['subscriberclicks'],
'unsubscribes': item['unsubscribes'],
'hardbounces': item['hardbounces'],
'softbounces': item['softbounces'],
}
int_camps_list.append(camp)
camps_list.extend(int_camps_list)
return camps_list
Summary
In this blog, we explored the ActiveCampaign API and its capabilities for retrieving data efficiently, with a focus on pagination.
By utilizing pagination parameters such as limit
, offset
, we can control the number of results per request and navigate through the data effectively.